This article details the basic syntax for defining constants, including libraries, making comments, and using punctuation in your Arduino programs.
Defining constants:
The #define syntax allows the programmer to give a name to a constant value before the program is compiled, allowing any references to the constant to be replaced with the defined value when the program is running.
However, although convenient, using #define presents a few things to watch out for:
Firstly, proper syntax:
When writing “#define constantName value”, you cannot punctuate the line with a semicolon (unlike other lines of code).
Additionally, you must be careful to not involuntarily include an “=” in between the constantName and the value that is being defined.
Const
Writing const before a variable in the code setup() or before will change the variable to a “read-only” format, meaning that its value cannot be changed later in the program. In other words, it is constant. It serves as a more popular alternative to #define.
When using arrays (a data type referencing a collection of variables), you cannot use const.
A benefit of using const over #define is that const allows you to specify a data type for the constant being defined, as you can see in this image:
In the following code, pi is declared as a float (a data type similar to an integer, except where decimals are included in the constant). For a challenge, try to identify the error in the code below:
--------
const float pi = 3.14;
float x;
// ....
x = pi*2;
pi = 7;
---------
The error: In the first line, pi is declared as a constant; for this reason, improper syntax is used in the last line, as it tries to modify the constant's value.
Variable Scope
Declaring variables and defining constants only becomes useful when they are declared in the right parts of a program; this is called variable scope, and it’s the idea that any variable is limited to the place in which it’s defined. For instance, when a variable/constant is declared outside both the setup and the loop functions, it is called a global variable, meaning it can be used throughout the program. On the other hand, if a variable is declared within a for-loop, it can only be used within that loop (if you try to reference a local variable in a segment of code where it was not originally defined, the program will return an undefined value).
Including Libraries:
#include<library.h>
Using #include allows programmer to use and reference premade libraries in their program.
When using an external component in your circuit, like a LCD display for instance, you would include that component’s premade library in your program so that the microcontroller would recognize it (this is a simplified explanation of how libraries work). Here is an example of what that looks like in practice:
In this image, take note of the syntax when referencing libraries:

In this video, take note of how I access the premade libraries by clicking "Tools" and then finding the "Manage Libraries" tab:
Writing Comments:
Comments
Comments are ignored by the processor and serve as a way to inform yourself and others about the way a program works.
Single-Line Comments //
These comments have no coding functionality, but merely allow you to add your own thoughts or reminders in the code. When using the // syntax, the comment cannot take up more than one line of code.
Block Comment /* */
Serve the same purpose as single-line comments, but using this syntax allows for a multi-line comment: the comment must start with /* and end with */. Everything in between these two symbols will be ignored by the compiler and will not occupy any space in your program.
Usually, I use a block comment when I need to modify an aspect of code or identify the aspect of a program that is causing a given error but not remove it entirely in the process; in these instances, I comment the original code and modify it outside of the comment.
In the below image, you can see that I have commented to remind myself which pin refers to which color of LED:
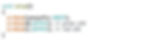
Punctuation:
Semicolon ;
Semicolons are used to end a statement (line of code).
Even if you are writing code within an if statement, you must always punctuate each line with a semicolon.
Curly Braces {}
The opening brace must be followed by a closing brace. Arduino IDE software packs a useful feature that allows you to check that the brackets align properly by clicking on (or near) one brace; once you do this, the paired brace will be highlighted.
These braces are used in:
Conditional statements
Loops
While
Do
For
Functions
All of these will be detailed in an upcoming article, which you will soon find linked in a comment on this post.
Here is an example of the braces in one of my programs:
